Sources
https://github.com/abhinavsagar/Machine-Learning-Deployment
https://towardsdatascience.com/how-to-easily-deploy-machine-learning-models-using-flask-b95af8fe34d4
Graphical user interface
https://github.com/abhinavsagar/Machine-Learning-Deployment
https://towardsdatascience.com/how-to-easily-deploy-machine-learning-models-using-flask-b95af8fe34d4
Project Structure
This project has four parts :
- model.py — This contains code for the machine learning model to predict sales in the third month based on the sales in the first two months.
- app.py — This contains Flask APIs that receives sales details through GUI or API calls, computes the predicted value based on our model and returns it.
- request.py — This uses requests module to call APIs defined in app.py and displays the returned value.
- HTML/CSS — This contains the HTML template and CSS styling to allow user to enter sales detail and displays the predicted sales in the third month.
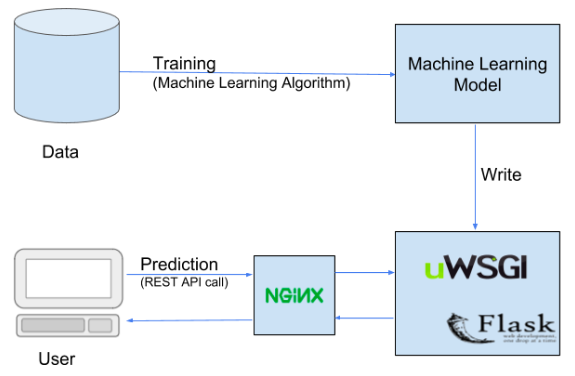
app.py
import numpy as np | |
from flask import Flask, request, jsonify, render_template | |
import pickle | |
app = Flask(__name__) | |
model = pickle.load(open('model.pkl', 'rb')) | |
@app.route('/') | |
def home(): | |
return render_template('index.html') | |
@app.route('/predict',methods=['POST']) | |
def predict(): | |
int_features = [int(x) for x in request.form.values()] | |
final_features = [np.array(int_features)] | |
prediction = model.predict(final_features) | |
output = round(prediction[0], 2) | |
return render_template('index.html', prediction_text='Sales should be $ {}'.format(output)) | |
@app.route('/results',methods=['POST']) | |
def results(): | |
data = request.get_json(force=True) | |
prediction = model.predict([np.array(list(data.values()))]) | |
output = prediction[0] | |
return jsonify(output) | |
if __name__ == "__main__": | |
app.run(debug=True)
request.py |
import requests | |
url = 'http://localhost:5000/results' | |
r = requests.post(url,json={'rate':5, 'sales_in_first_month':200, 'sales_in_second_month':400}) | |
print(r.json()) |
Results
Run the web application using this command.
$ python app.py
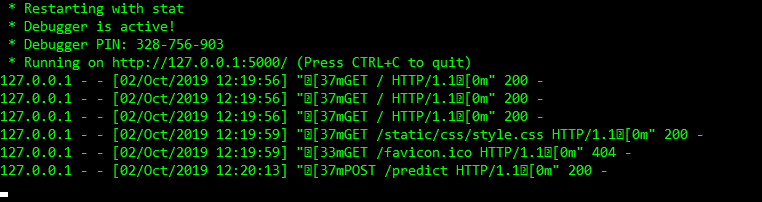
Open http://127.0.0.1:5000/ in your web-browser, and the GUI as shown below should appear.
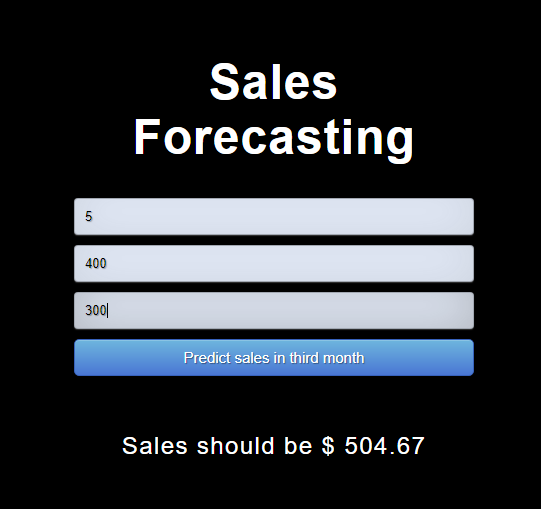
No comments:
Post a Comment